리액트 라이프사이클
리액트 라이프사이클 연습 예제 : 로직 진행순서 맞추기
0231
1번: useEffect의 dependency array가 빈배열로 들어와있다는 것은 mount가 되었을 때 실행하라는 뜻 = mount가 되었다는 것은 코드 top~bottom 까지 랜더링 1회가 다 되고 난 후를 뜻함
0231(리랜더링)023
1번 : useEffect 로 들어와서 setA(2)로 state값이 변경이 되었기 때문에 다시 리랜더링 시작(top~bottom)
그리고 나서 마지막 3번을 실행한 후, useEffect로 올라가지 않고 마무리(why? dependency array가 빈배열이기때문에, state 값이 바뀐것도 아니기에)
0231(리랜더링)023
무한루프
axios
(1) axios 터미널에 설치하기
yarn add axios
(2) axios 를 사용하는 이유
axios 를 사용하면 첫번째 then. 을 생략할 수 있다.
(응답 데이터를 JSON 형식으로 파싱 순서를 뛰어넘을 수 있다)
따라서 더 직관적이고, 편리하다.
fetch('https://jsonplaceholder.typicode.com/todos/1')
.then(response => response.json())
.then(json => console.log(json))
=>
axios.get('주소')
.then(function(response){
console.log(response.data);
})
(3) axios 를 사용해 외부 API 가져오기
- 데이터를 저장해줄 state 만들기(선언)
- response.data 를 state에 담아주기
- 화면에 보일 수 있도록 map 으로 데이터 뿌려주기
기본 구조 (1~2번 과정 해당)
import React, { useEffect } from "react";
import axios from "axios";
function App() {
useEffect(()=>{
axios
.get('https://jsonplaceholder.typicode.com/todos')
.then(function(response){
console.log(response);
})
.catch(function(error){
console.log("error!");
console.log(error);
});
},[])
return (
<>
<div>hello</div>
</>
);
}
export default App;
데이터 화면에 랜더링하기 (1~3번 과정 해당)
1. Axios + then
import React, { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [lists, setLists] = useState([]);
useEffect(()=>{
axios
.get('https://jsonplaceholder.typicode.com/todos')
.then(function(response){
console.log(response.data);
setLists([...response.data]);
})
.catch(function(error){
console.log("error!");
console.log(error);
});
},[])
return (
<>
{lists.map(function(list){
return(
<ul key={list.id}>
<li>userID:{list.userId}</li>
<li>title:{list.title}</li>
</ul>
);
})}
</>
);
}
export default App;
2. Axios + async / await
import React, { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [lists, setLists] = useState([]);
useEffect(()=>{
const getList = async()=> {
const response = await axios.get('https://jsonplaceholder.typicode.com/todos');
setLists(response.data);
}
getList();
},[])
return (
<>
{lists.map(function(list){
return(
<ul key={list.id}>
<li>userID:{list.userId}</li>
<li>title:{list.title}</li>
</ul>
);
})}
</>
);
}
export default App;
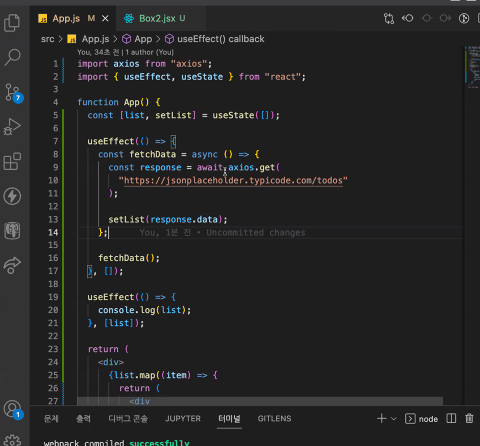
2-1. Axios + async / await + loading state 도 추가
import React, { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [lists, setLists] = useState([]);
const [isLoading, setIsLoading] = useState(false);
useEffect(()=>{
const getList = async()=> {
setIsLoading(true);
const response = await axios.get('https://jsonplaceholder.typicode.com/todos');
setIsLoading(false);
setLists(response.data);
}
getList();
},[])
if (isLoading === true) {
return <div>Loading~~~</div>
}
return (
<>
{isLoading === false &&
lists.map(function(list){
return(
<ul key={list.id}>
<li>userID:{list.userId}</li>
<li>title:{list.title}</li>
</ul>
);
})
}
{isLoading === true && <div>로딩중...</div>}
</>
);
}
export default App;
isError, isLoading 은 필수!!!!
데이터요청 전, isloading true / isError false
데이터 응답 받아온 후,
- 응답 제대로 받아온 경우: isloading false / isError false
- 응답이 제대로 안온경우: isloading false / isError true
나중에는 react query 라이브러리로 관리하여 쉽게 관리할 수 있지만, 위 예제로 개념을 알고 있어야 한다.
Axios + then + loading / error
import axios from "axios";
import { useEffect, useState } from "react";
function App() {
const [list, setList] = useState([]);
const [isLoading, setIsLoading] = useState(false);
const [isError, setIsError] = useState(false);
useEffect(() => {
setIsLoading(true);
setIsError(false);
axios
.get("https://jsonplacehlder.typicode.com/todos")
.then(function (response) {
// 정상응답
setIsLoading(false);
setIsError(false);
setList(response.data);
})
.catch(function (error) {
setIsLoading(false);
setIsError(true);
});
}, []);
useEffect(() => {
console.log(list);
}, [list]);
return (
<div>
<h1>List</h1>
{isLoading === true && <div>로딩중...</div>}
{isError === true && <div>오류가 발생하였습니다.</div>}
{isLoading === false &&
list.map((item) => {
return (
<div
style={{
padding: "10px",
}}
key={item.id}
>
{item.title}
</div>
);
})}
</div>
);
}
export default App;
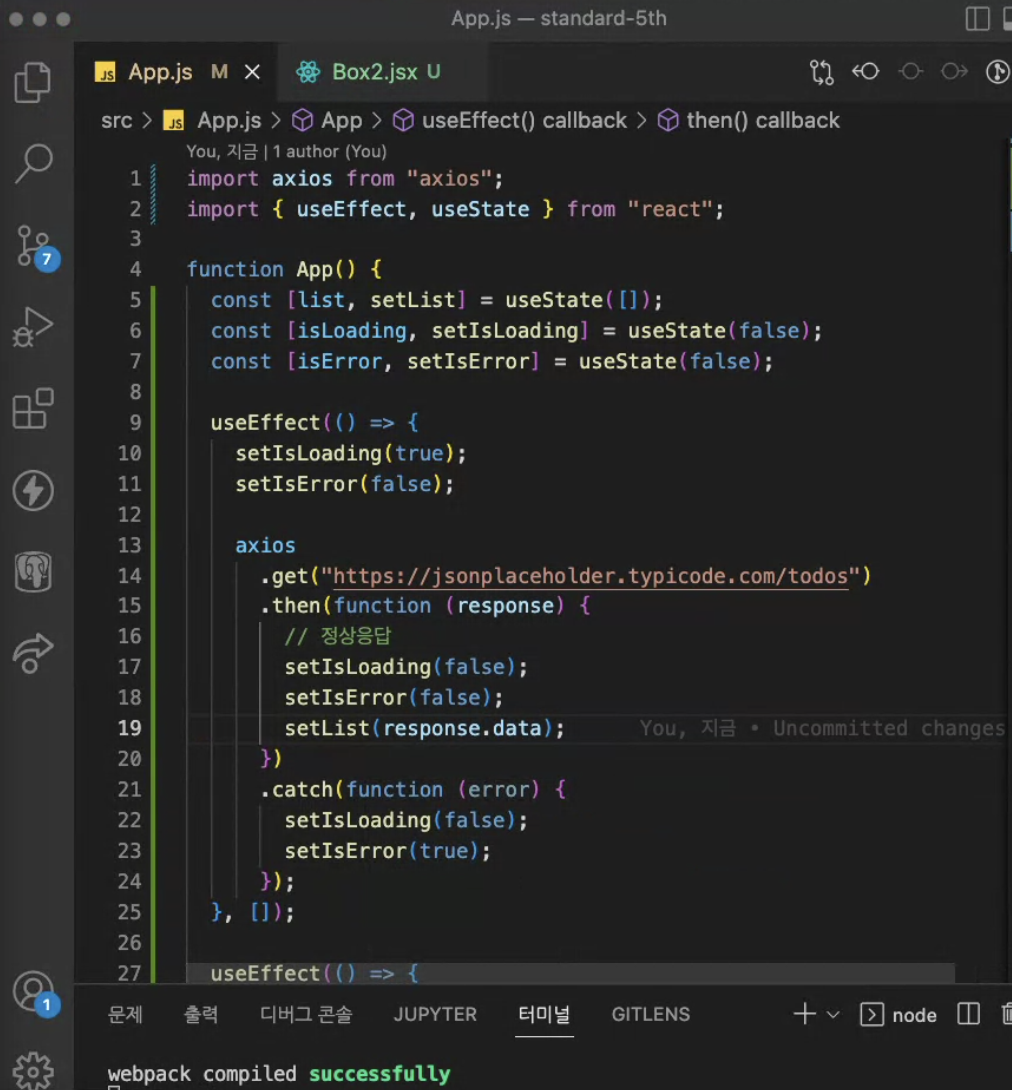
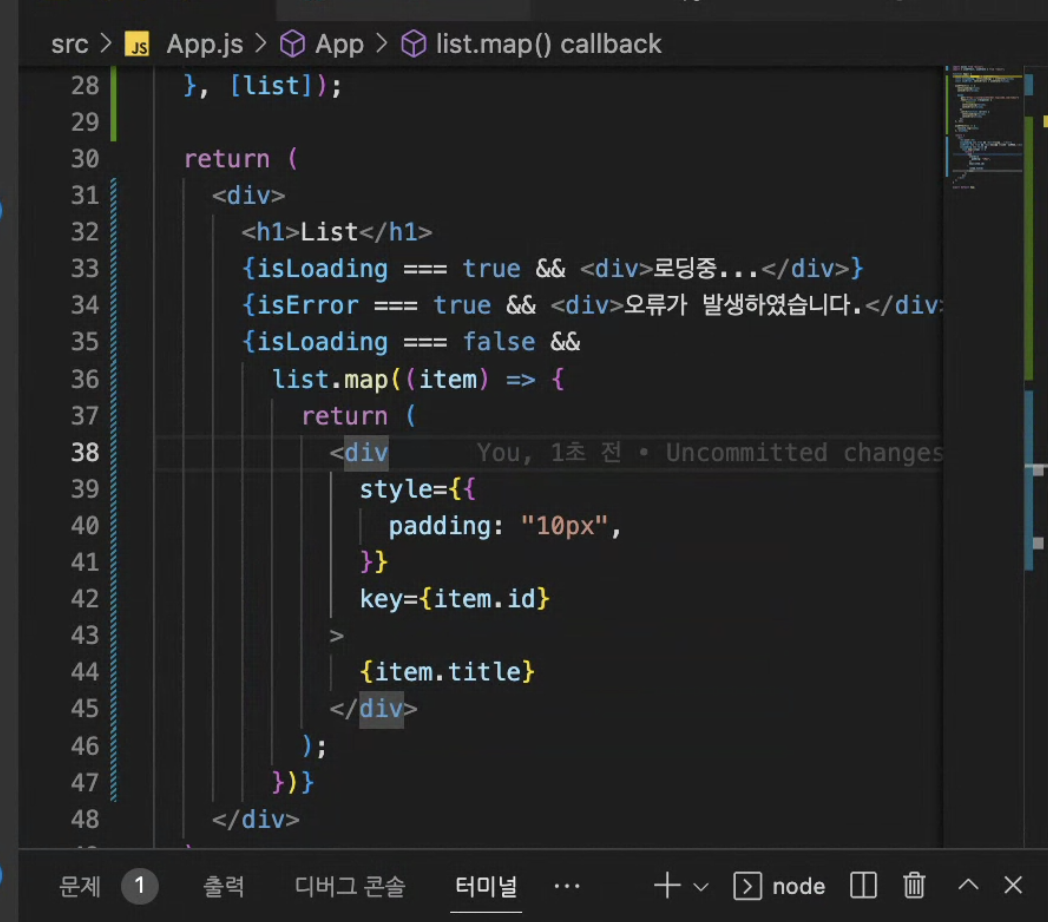
'부트캠프 개발일지 2023-2024 > Bootcamp 생활기록' 카테고리의 다른 글
[8주차] 리액트: 그룹 프로젝트 newsfeed, 음악 커뮤니티 만들기 (0) | 2023.11.21 |
---|---|
[7주차] 리액트: 커서 위치 맨 마지막으로 설정하기(setSelectionRange) (0) | 2023.11.17 |
[7주차] 리액트: json 데이터를 서로다른 페이지에서 모두 받아야하는 경우 (0) | 2023.11.16 |
[7주차] 리액트: 확인/취소 알림창 (0) | 2023.11.16 |
[7주차] 리액트: CSS 초기설정 중 중요한 것들 (0) | 2023.11.16 |