Redux 설치
1. 리액트 프로젝트 새로 만들기
2. 터미널을 열어 리덕스 설치
yarn add redux react-redux
또는
yarn add redux
yarn add react-redux
**react-redux 는 패키지는 리덕스를 리액트에서 사용할 수 있도록 서로 연결시켜주는 패키지
3. 폴더구조 생성하기
- src > redux 폴더생성
- redux > config, modules 폴더생성
- redux : 리덕스와 관련된 코드를 모두 모아 놓을 폴더
- config : 리덕스 설정과 관련된 파일들을 놓을 폴더
- configStore : “중앙 state 관리소" 인 Store를 만드는 설정 코드들이 있는 파일
- modules : 우리가 만들 State들의 그룹이라고 생각하면 된다. 예를 들어 `투두리스트`를 만든다고 한다면, 투두리스트에 필요한 `state`들이 모두 모여있을 `todos.js`를 생성하게 되는데 이 `todos.js` 파일이 곧 하나의 모듈이 되는 원리이다.
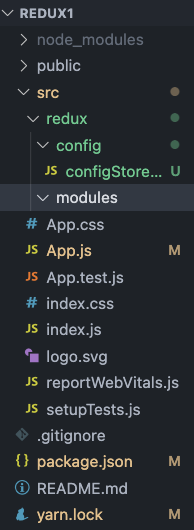
Redux 초기설정
4. 설정코드 작성
src/config/configStore.js 에 아래 코드를 입력하기
import { createStore } from "redux";
import { combineReducers } from "redux";
/*
1. createStore()
리덕스의 가장 핵심이 되는 스토어를 만드는 메소드(함수) 입니다.
리덕스는 단일 스토어로 모든 상태 트리를 관리한다고 설명해 드렸죠?
리덕스를 사용할 시 creatorStore를 호출할 일은 한 번밖에 없을 거예요.
*/
/*
2. combineReducers()
리덕스는 action —> dispatch —> reducer 순으로 동작한다고 말씀드렸죠?
이때 애플리케이션이 복잡해지게 되면 reducer 부분을 여러 개로 나눠야 하는 경우가 발생합니다.
combineReducers은 여러 개의 독립적인 reducer의 반환 값을 하나의 상태 객체로 만들어줍니다.
*/
const rootReducer = combineReducers({});
const store = createStore(rootReducer);
export default store;
디렉토리 최상단의 index.js 안에 아래 내용 입력
// 원래부터 있던 코드
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
// 우리가 추가할 코드
import store from "./redux/config/configStore";
import { Provider } from "react-redux";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
//App을 Provider로 감싸주고, configStore에서 export default 한 store를 넣어줍니다.
<Provider store={store}>
<App />
</Provider>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
++ 리덕스 작동원리
리덕스 작동 원리
action —> dispatch —> reducer 순으로 동작
action: 앱에서 store로 운반할 데이터, 즉 상태 변화를 담은 객체이다. (state 변경 함수)
dispatch: '보내다'라는 뜻인데, dispatch 안에 사용할 action을 넣어서 이걸 reducer로 보내주는 것
reducer: action이 reducer 라는 함수로 전달이 되고 이 함수가 넘겨 받은 action 을 가지고 새로운 state를 만든다. 그걸 다시 store 로 넘겨줘서 화면에 보여지게 되는 것이다.
리액트 Redux reducer 사용법
useState는 state값을 변경할 때 1) state의 초기값을 설정해주고 2) 그걸 state 변경 함수를 통해서 값을 바꿔주는 방식이었다. Redux 에서는 어떻게 state를 변경할 수 있을까? 🔍 reducer를 이용하여 수정
velog.io
Redux state에 대한 초기설정 식
src > redux > modules > counter.js
현재 counter.js 에는 counter 에 대한 state들이 모여 있다
1.모듈 파일에서 초기 상태값 설정하기 (초기값은 배열,객체,원시데이터 모두 가능)
// counter.js
const initialState = {
number : 0,
};
일반 버전에서의 state 선언식과 같은 역할을 한다. const [number, setNumber] = useState(0) 와 같다.
2. 리듀서 작성하기 (리듀서는 변화를 일으키는 함수)
기본형 const counter = (state, action) => {}
action 은 상태변화를 담은 객체, 리듀서는 state를 action의 type에 따라 변경하는 함수
// counter.js
const counter = (state = initialState, action) => {
switch (action.type){
default:
return state;
}
}
export default counter;
3. counter 모듈을 스토어에 연결
counte에 대한 import 하기
configStore.js 의 const rootReducer = combineReducers({})의 객체 안에 Key-value 넣기
counter:counter 을 넣었다.
const rootReducer = combineReducers({
counter: counter,
});
전체식
//src>reduex>config>configStore.js
import {createStore} from "redux";
import {combineReducers} from "redux";
import counter from "../modules/counter";
const rootReducer = combineReducers({
counter: counter,
});
const store = createStore(rootReducer);
export default store;
4. store 와 module 연결확인하기
useSelector 를 이용해 스토어 조회하기
- App.js 으로 이동한다
- App.js 에서 store에 접근하여 counter의 값을 읽고싶을 때 또는 useSelector 를 이용해 스토어 조회하기 위해서 useSelector 를 사용한다
- useSelector 사용시, import 잊지 않고 해야한다
**여기서의 state 는 store의 전체 state
**이 전체 state가 어떤 건지 콘솔로 한번 찍어서 확인해본다
const data = useSelector((state)=> {
return state;
});
console.log('data',data);
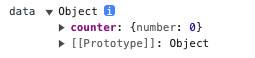
import logo from './logo.svg';
import './App.css';
import { useSelector } from 'react-redux';
function App() {
// App.js 에서 store에 접근하여 counter의 값을 읽고싶을 때
// 여기서의 state 는 store의 전체 state
const data = useSelector((state)=> {
return state;
});
console.log('data',data); // data = { counter: {number: 0}, ... }
return (
<div className="App">
Redux
</div>
);
}
export default App;
여기서 이제!
나는 counter의 data만 가져오고 싶다면 어떻게 해야할까?
const counter = useSelector((state)=> {
return state.counter;
});
console.log(counter);
// counter = {number:0}
더 나아가 counter의 value 값(객체)의 value값을 가져오고 싶다면?
const counter = useSelector((state)=> {
return state.counter;
});
console.log(counter.number);
// counter = 0
또는
const counter = useSelector((state)=> {
return state.counter;
});
console.log(counter.number);
// counter = {number:0}
'부트캠프 개발일지 2023-2024 > React 리액트' 카테고리의 다른 글
[6주차] 리액트 스탠다드 수업: 조건부 랜더링 (매우중요) (0) | 2023.11.10 |
---|---|
[6주차] 리액트숙련: Redux 로 카운터 만들기 - useDispatch (0) | 2023.11.09 |
[6주차] 리액트숙련: Redux 소개 (0) | 2023.11.09 |
[6주차] 리액트숙련: 가상DOM (Virtual DOM) (0) | 2023.11.09 |
[6주차] 리액트숙련: React Hooks - 최적화(React.memo, useCallback, useMemo) (0) | 2023.11.08 |